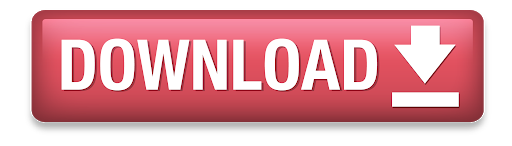
- Ruby Programming For Kids
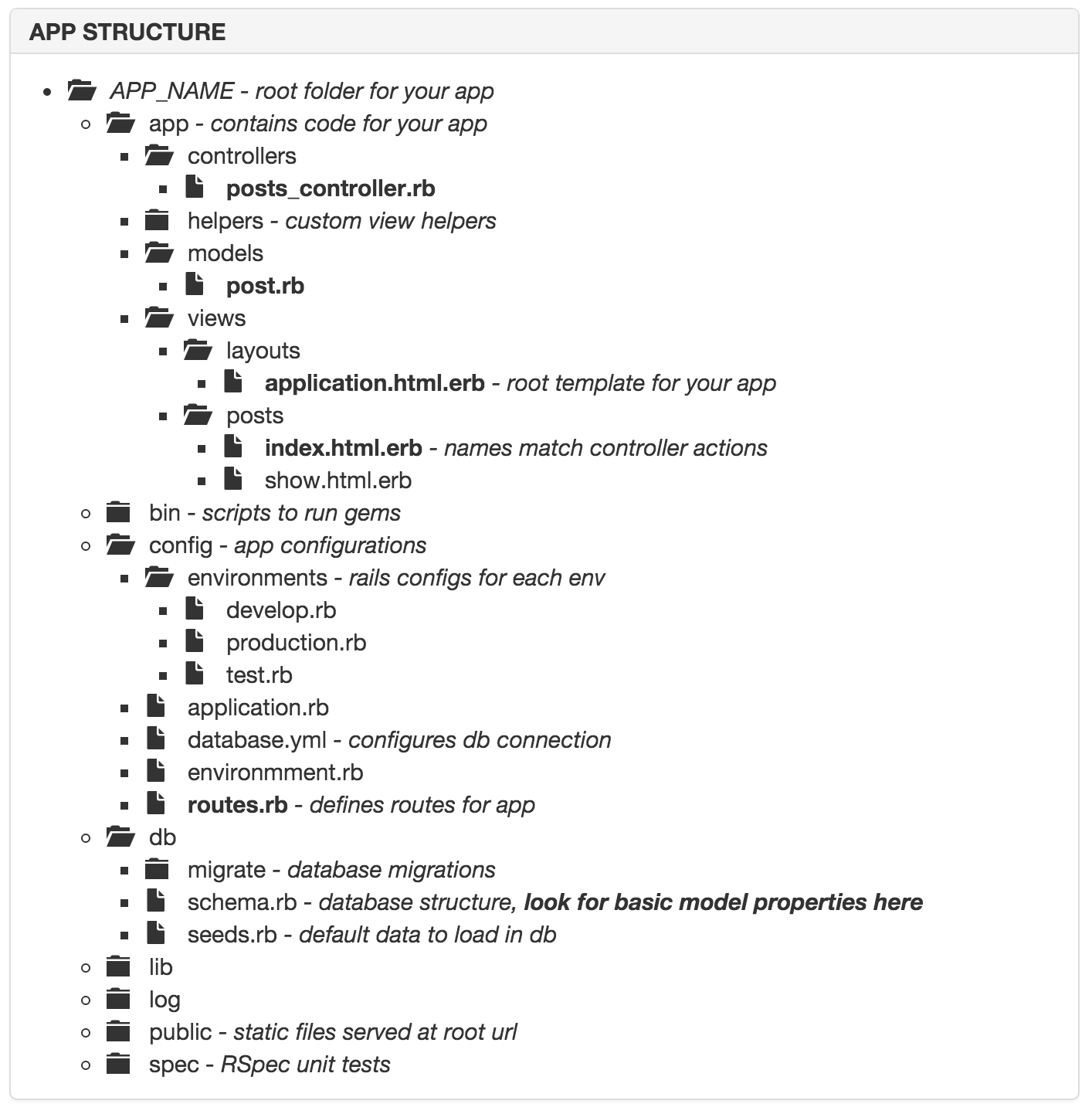
Please be warned, applying cheats in a Pokemon Ruby game is fun but sometimes cheats can ruin a game. The sad fact is that, cheats aren’t support on any Pokemon games. C-media hard disk controller driver. Save your game before apply cheats, when glitches occur like bad egg, don’t save your game. When freezing or random crashing happens, restart your emulator. Ruby Language Cheat Sheet We provide you with all the mostly used Ruby modules and corresponding function and feature list. You can click on the feature and see more details about the feature with example code. Navman nz driver download. We hope you will enjoy the cheat sheet and developer hints very much.
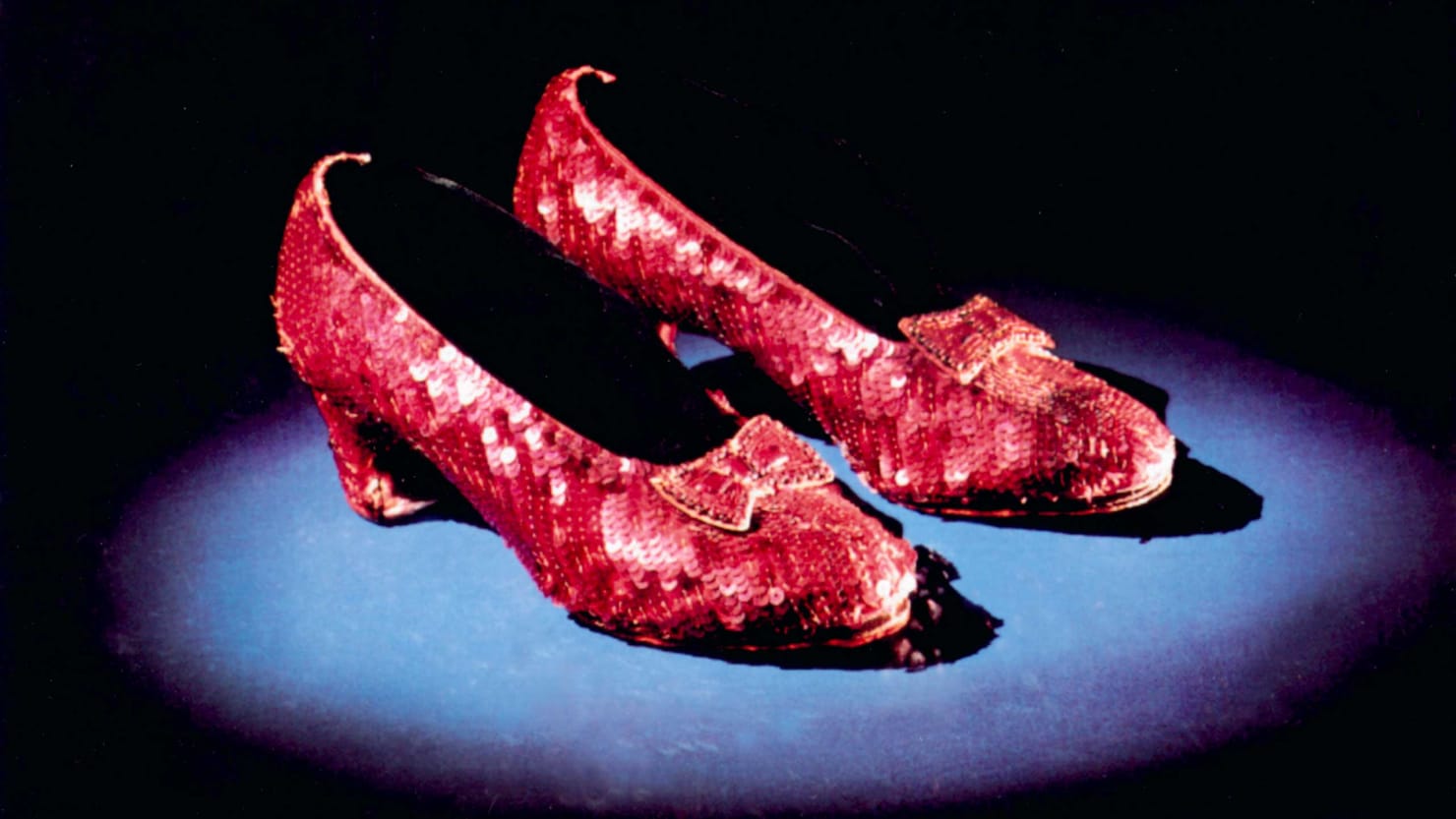
Ruby Programming For Kids
Ruby Cheat Sheet# Ruby code reads a lot like English, which is good. # But its syntax is a little weird looking if you're used # to other common languages like C++, Javascript, Java, etc.# (Or really, really weird if you've never programmed before!)
# Comments look like this, (with a pound symbol at the front of the line.) # Commented lines are ignored. # Ruby variables are pretty straightforward. # They can contain any type. Let's create some variables. a = 'Some string' b = 5 # an integer c = 5 + 5 d = c * b # d now contains 50, right? e = 10.0 # a float is_awesome = true # a boolean another_string = 'This one has 'quotes' my_point = Geom::Point3d.new(10, 0, 0) # an object provided by SketchUp # Strings can be concatenated, like so.. first_name = 'John' last_name = 'Doe' full_name = first_name + ' ' + last_name # But to concatenate strings to non-strings, # you must 'cast' them, with the to_s method. age = 25 sentence = full_name + ' is ' + age.to_s + ' years old.' # There are also methods for converting to float and integer. my_float = 5.to_f my_integer = (5.0 + 10.0).to_i my_other_integer = '5'.to_i # Methods can be called with or without parentheses. # puts() prints to the console. puts('Hello world!') puts 'Hello world!' # Methods are defined with the def/end/return keywords. You # can put default values onto parameters with the = declaration def do_square(val, return_integer=false) my_float_value = val.to_f square = my_float_value * my_float_value if return_integer true return square.to_i else return square end end puts (do_square(5)) # Will print out 25.0 puts do_square(3.1, true) # Will print 9 # Arrays are declared with [] and have lots of handy methods. languages = ['Ruby', 'English', 'SP']
languages.reverse puts languages.first # 'SP' puts languages[1] # English last_index = languages.length - 1 puts languages[last_index] # 'Ruby' puts languages.last # 'French'
# Hashes are declared with {}, and can be initialized with # values via the => operator. Both dot syntax and array access # by key works. hobbies = { 'scott'=>'games', 'tyler'=>'bikes', 'simone'=>'futbal' } puts hobbies.scott # 'games' puts hobbies['tyler'] # 'bikes' that_italian_guy = 'simone' puts(hobbies[that_italian_guy]) # 'futbal' hobbies.tyson = 'Carpentry' hobbies['John'] = 'Skiing' # Arrays (and most every kind of object) have this neat .each method. languages.each { |lang| puts 'My favorite language is ' + lang } languages.each { |lang| puts 'My favorite language is ' + lang } hobbies.each { |first_name, hobby| puts first_name + ' likes ' + hobby } |
|